Algorithms and Data Structures in Swift
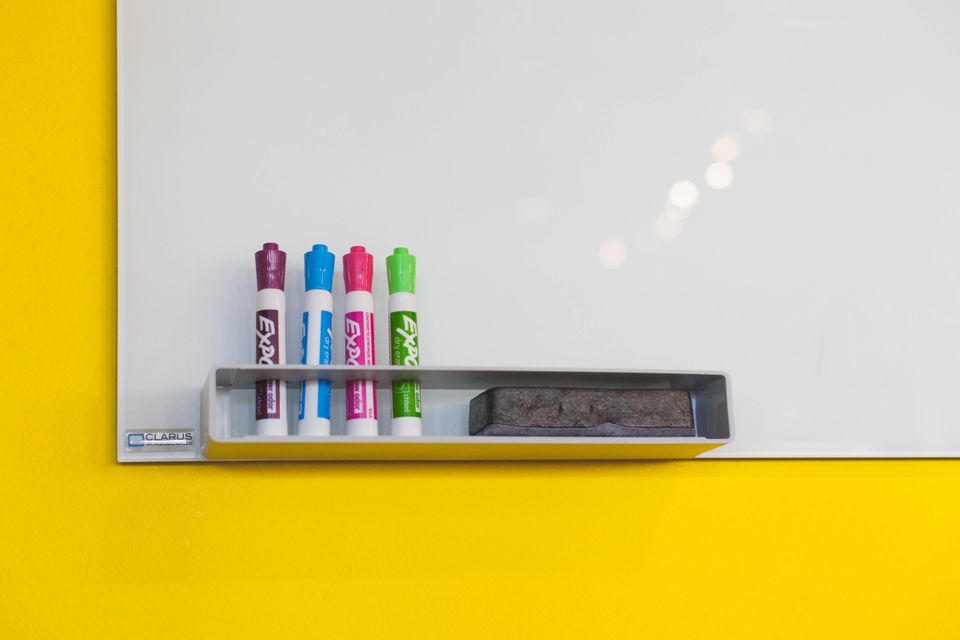
So you’ve read Apple’s Swift language docs, experimented in bunch of Swift Playgrounds, and have successfully built some small iOS apps…what’s next? In the forthcoming series of posts I intend to answer this question by teaching the basic concepts of algorithms and data structures, using examples written in Swift. After you’ve gotten a good feel of the language and the basics of iOS app development, it’s important to focus on writing more efficient code. To be clear, when I use the word ‘efficient’ I’m talking about how well your iOS app is using its host device’s resources to get a particular job done. You can think about this in terms of space and time, i.e. how much storage space on disk do you need and how long does your code take to run?
Think about efficiency in terms of painting your room:
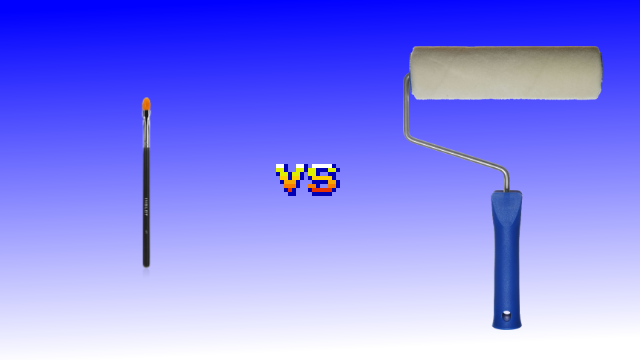
You could take a slow methodical approach (i.e. using your paint brush), but there is often a faster method that accomplishes the same goal by reducing repetition.
We can describe the efficiency of code using something called Big O Notation. Every time you use this notation you literally write a capital O, parenthesis, and some algebraic expression in the parenthesis. The algebraic expression is always going to be a mathematical function of the variable n, where n represents the length of an input into your function.
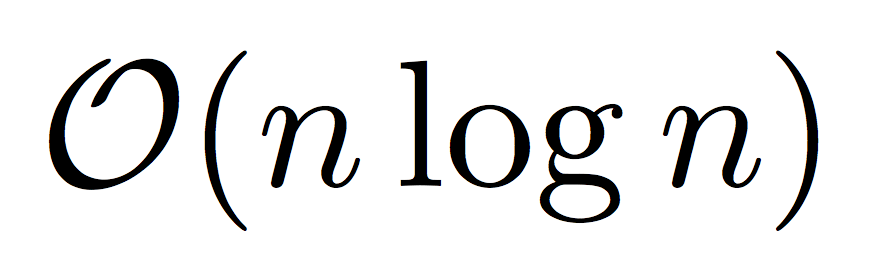
In a technical interview you will be expected to describe the efficiency of your solution. Fortunately, Big O Notation provides us with the means of articulating it. In the next blog post, I’ll go into more depth about Big O Notation and make this concept seem less foreign!
Member discussion